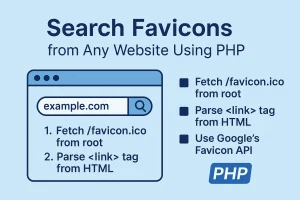
Introduction
Ever wondered how to automatically extract a website’s favicon using PHP? Whether you’re building a bookmark manager, a web scraper, or a browser-like dashboard, retrieving favicons is a great way to enhance user experience and add a visual touch.
This guide shows you how to search and fetch favicons from any website using PHP. We’ll cover techniques ranging from simple HTML parsing to fallback options using public APIs.
What Is a Favicon?
A favicon is a small icon that represents a website. You usually see it on:
-
Browser tabs
-
Bookmarks
-
Mobile browser previews
-
Custom dashboards and web UIs
The favicon is usually stored at:
https://example.com/favicon.ico
However, many modern sites use <link rel="icon" />
or load favicons dynamically, which means a reliable PHP solution must handle both default locations and custom paths.
Why Use PHP to Fetch Favicons?
You may need favicon fetching in:
-
Bookmark Managers
-
CMS Plugins or Dashboards
-
SEO or web analytics tools
-
Browser emulators or preview generators
Using PHP to fetch favicons makes it easy to automate the process, store icons, or display them in your UI.
Method 1: Fetch Default Favicon from Root
This is the simplest method. It assumes the favicon is located at /favicon.ico
.
✅ PHP Code:
function getDefaultFavicon($url) {
$parsedUrl = parse_url($url);
$host = $parsedUrl['scheme'] . "://" . $parsedUrl['host'];
return $host . "/favicon.ico";
}
// Example:
echo getDefaultFavicon("https://www.google.com");
🔍 Limitation:
Not all sites store the favicon at /favicon.ico
. Some use custom paths defined in HTML.
Method 2: Parse <link rel="icon">
Tag from HTML
A more robust solution is to fetch the HTML of the page and extract the favicon path.
🧠 PHP Code Example:
function getFaviconFromHTML($url) {
$html = @file_get_contents($url);
if (!$html) return false;
preg_match_all('/<link[^>]+rel=["\']?(shortcut icon|icon)["\']?[^>]*>/i', $html, $matches);
foreach ($matches[0] as $linkTag) {
if (preg_match('/href=["\']?([^"\']+)["\']?/', $linkTag, $hrefMatch)) {
$favicon = $hrefMatch[1];
if (strpos($favicon, 'http') !== 0) {
// Convert relative path to absolute
$parsedUrl = parse_url($url);
$base = $parsedUrl['scheme'] . '://' . $parsedUrl['host'];
return $base . '/' . ltrim($favicon, '/');
}
return $favicon;
}
}
return getDefaultFavicon($url);
}
🧪 Output:
echo getFaviconFromHTML("https://www.github.com");
// Returns full path to favicon
Method 3: Use Google’s Favicon API (Fast & Reliable)
If you want a quick and reliable solution without HTML parsing or URL headaches, use Google’s service:
🧩 PHP Snippet:
function getFaviconFromGoogle($url) {
$parsed = parse_url($url);
$domain = $parsed['host'];
return "https://www.google.com/s2/favicons?domain=" . $domain;
}
🌟 Benefits:
-
Fast loading
-
No HTML parsing
-
Works for most domains
⚠️ Note:
-
It fetches 16×16 size icons only
-
May not support non-public domains
Method 4: Use External APIs (Advanced)
There are also commercial APIs for more control and sizes:
Example with Clearbit:
function getFaviconFromClearbit($url) {
$parsed = parse_url($url);
return "https://logo.clearbit.com/" . $parsed['host'];
}
Caching Favicons for Performance
To reduce repeated requests and API limits, save the favicon locally.
🗂️ Example Code:
function saveFaviconLocally($faviconUrl, $localPath) {
$icon = file_get_contents($faviconUrl);
file_put_contents($localPath, $icon);
}
Common Errors & Fixes
Issue | Fix |
---|---|
Favicon not found | Fallback to root /favicon.ico or Google API |
file_get_contents fails |
Enable allow_url_fopen in php.ini |
Slow loading | Cache icons locally |
Relative favicon path | Convert to absolute using domain name |
Best Practices
-
Always validate the favicon URL using
filter_var()
-
Use HTTPS URLs to avoid mixed content warnings
-
Cache favicons using file naming based on domain hashes
-
Test on mobile and desktop for icon visibility
FAQ
Q: Can I use CURL instead of file_get_contents()?
Yes, CURL offers better error handling and header control.
Q: Will this work for HTTPS and HTTP sites?
Yes, as long as your server can access the page and favicon location.
Q: Can I get high-resolution favicons (e.g., 64×64)?
Use APIs like Clearbit or DuckDuckGo that support scalable icons.
Conclusion
Fetching favicons from websites using PHP is a small yet powerful feature that can enhance your app’s UX. Whether you’re working on a browser UI, web scraper, or internal dashboard, this guide helps you choose the right method—be it raw HTML parsing, default paths, or Google’s Favicon API.
Call to Action
🧑💻 Want help building your custom PHP application or API integration?
📞 Contact Sadedar Digital Solutions to automate data tasks, build scrapers, or enhance your backend with expert PHP support.